Intercept Screens Actions with the Paywall Action Interceptor
This section provides a details overview of the Paywall Action Interceptor and how to intercept actions
What is the Paywall Action Interceptor?
The Paywall Action Interceptor allows to intercept and override every interaction the users have with a Purchasely Screen.
This can be used to:
- Intercept purchase and restore actions to perform them using your own code or another third-party SDK
- Intercept the login button tapped to display your login form
- Force the explicit acceptance of terms and conditions before a purchase
- Intercept the call to a webview to inject credentials and be directly logged in
- Block purchases in Kids category apps to add a parental permission gate
- Block direct access to external content (webview or link to Safari) in Kids category apps to add a parental permission gate
With the action interceptor, you get everything you need to:
- Get the action (purchase, login, ...) and context (Plan purchased for instance)
- Display views, errors, messages, … above the Purchasely Screens
- Choose if Purchasely should continue the action or not
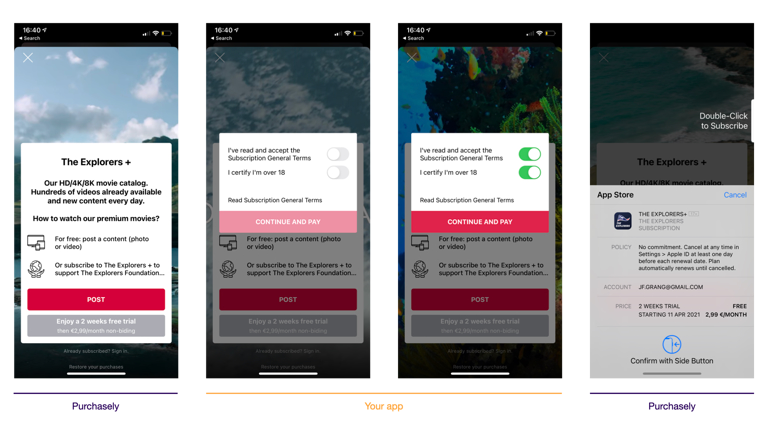
Example of use of the Paywall Action Interceptor: when a user clicks on the Purchase button, the SDK hands over to the app that displays a modal to make the user accept the T&C. The same principle is used to make the app process the transaction with an already-in-place transaction infrastructure
What Paywall Actions can be intercepted?
You can intercept the following buttons being tapped:
- Close
- Login
- Navigate (web or deeplink)
- Purchase
- Win-back / retention offer
- Restore
- Open another paywall
- Promo code
Intercepting an action
Here is a sample code to show how to intercept a login or to make the user accept terms & conditions before proceeding to the purchase.
Note: This mechanism can also be used in full
mode.
Purchasely.setPaywallActionsInterceptor { [weak self] (action, parameters, info, proceed) in
switch action {
// Intercept the tap on login
case .login:
// When the user has completed the process
// Pass true to reload the paywall if user is logged in
self?.presentLogin(above: info?.controller) { (loggedIn) in
Purchasely.userLogin(with: "MY_USER_ID")
proceed(loggedIn)
}
// Intercept the tap on purchase to display the terms and condition
case .purchase:
self?.presentTermsAndConditions(above: info?.controller) { (userAcceptedTerms) in
proceed(userAcceptedTerms)
}
default:
proceed(true)
break
}
}
Purchasely.setPaywallActionsInterceptor { info, action, parameters, processAction ->
if (info?.activity == null) return@setPaywallActionsInterceptor
when(action) {
PLYPresentationAction.PURCHASE -> {
presentTermsAndConditions(info.activity) { userAcceptedTerms ->
// Don't forget to notify the SDK by calling `processAction`
processAction(userAcceptedTerms)
}
PLYPresentationAction.LOGIN -> {
// Call your method to display your view
// and return boolean result to userLoggedIn
presentLogin(info.activity) { userLoggedIn ->
Purchasely.userLogin("MY_USER_ID")
// Pass true to reload the paywall if user is logged in
processAction(userLoggedIn)
}
}
else -> {
Log.d("PLYActionInterceptor", action.value + " " + parameters)
processAction(true)
}
}
}
Purchasely.setPaywallActionInterceptorCallback((result) => {
console.log('Received action from paywall ' + result.info.presentationId);
if (result.action === PLYPaywallAction.NAVIGATE) {
console.log(
'User wants to navigate to website ' +
result.parameters.title +
' ' +
result.parameters.url
);
Purchasely.onProcessAction(true);
} else if (result.action === PLYPaywallAction.CLOSE) {
console.log('User wants to close paywall');
Purchasely.onProcessAction(true);
} else if (result.action === PLYPaywallAction.LOGIN) {
console.log('User wants to login');
//Present your own screen for user to log in
Purchasely.closePresentation();
Purchasely.userLogin('MY_USER_ID');
//Call this method to update Purchasely Paywall
Purchasely.onProcessAction(true);
} else if (result.action === PLYPaywallAction.OPEN_PRESENTATION) {
console.log('User wants to open a new paywall');
Purchasely.onProcessAction(true);
} else if (result.action === PLYPaywallAction.PURCHASE) {
console.log('User wants to purchase');
//If you want to intercept it, close presentation and display your screen
Purchasely.closePresentation();
} else if (result.action === PLYPaywallAction.RESTORE) {
console.log('User wants to restore his purchases');
Purchasely.onProcessAction(true);
} else {
console.log('Action unknown ' + result.action);
Purchasely.onProcessAction(true);
}
});
Purchasely.setPaywallActionInterceptorCallback(
(PaywallActionInterceptorResult result) {
if (result.action == PLYPaywallAction.navigate) {
print('User wants to navigate');
Purchasely.onProcessAction(true);
} else if (result.action == PLYPaywallAction.close) {
print('User wants to close paywall');
Purchasely.onProcessAction(false);
} else if (result.action == PLYPaywallAction.login) {
print('User wants to login');
//Present your own screen for user to log in
Purchasely.closePresentation();
Purchasely.userLogin('MY_USER_ID');
//Call this method to update Purchasely Paywall
Purchasely.onProcessAction(true);
} else if (result.action == PLYPaywallAction.open_presentation) {
print('User wants to open a new paywall');
Purchasely.onProcessAction(true);
} else if (result.action == PLYPaywallAction.purchase) {
print('User wants to purchase');
//If you want to intercept it, close presentation and display your screen
Purchasely.closePresentation();
Purchasely.onProcessAction(false);
} else if (result.action == PLYPaywallAction.restore) {
print('User wants to restore his purchases');
Purchasely.onProcessAction(true);
} else {
print('Action unknown ' + result.action.toString());
Purchasely.onProcessAction(true);
}
});
Purchasely.setPaywallActionInterceptor((result) => {
console.log('Received action from paywall' + result.info.presentationId);
if (result.action === Purchasely.PaywallAction.navigate) {
console.log(
'User wants to navigate to website ' +
result.parameters.title +
' ' +
result.parameters.url
);
Purchasely.onProcessAction(true);
} else if (result.action === Purchasely.PaywallAction.close) {
console.log('User wants to close paywall');
Purchasely.onProcessAction(true);
} else if (result.action === Purchasely.PaywallAction.login) {
console.log('User wants to login');
//Present your own screen for user to log in
Purchasely.closePresentation();
Purchasely.userLogin('MY_USER_ID');
//Call this method to update Purchasely Paywall
Purchasely.onProcessAction(true);
} else if (result.action === Purchasely.PaywallAction.open_presentation) {
console.log('User wants to open a new paywall');
Purchasely.onProcessAction(true);
} else if (result.action === Purchasely.PaywallAction.purchase) {
console.log('User wants to purchase');
//If you want to intercept it, close presentation and display your screen
Purchasely.closePresentation();
} else if (result.action === Purchasely.PaywallAction.restore) {
console.log('User wants to restore his purchases');
Purchasely.onProcessAction(true);
} else {
console.log('Action unknown ' + result.action);
Purchasely.onProcessAction(true);
}
});
private PurchaselyRuntime.Purchasely _purchasely;
purchasely.SetPaywallActionInterceptor(OnPaywallActionIntercepted);
private void OnPaywallActionIntercepted(PaywallAction action)
{
Log($"Purchasely Paywall Action Intercepted. Action: {action.action}.");
switch (action.action)
{
case "navigate":
Log("User wants to navigate");
purchasely.ProcessPaywallAction(true);
break;
case "close":
Log("User wants to close paywall");
purchasely.ProcessPaywallAction(false);
break;
case "login":
Log("User wants to login");
// Present your own screen for user to log in
purchasely.ClosePresentation();
purchasely.UserLogin("MY_USER_ID");
// Call this method to update Purchasely Paywall
purchasely.ProcessPaywallAction(true);
break;
case "open_presentation":
Log("User wants to open a new presentation");
purchasely.ProcessPaywallAction(true);
break;
case "purchase":
Log("User wants to purchase");
// If you want to intercept it, close presentation and display your screen
purchasely.ClosePresentation();
purchasely.ProcessPaywallAction(false);
break;
case "restore":
Log("User wants to restore his purchases");
purchasely.ProcessPaywallAction(true);
break;
default:
Log($"Action unknown {action.action}");
purchasely.ProcessPaywallAction(true);
break;
}
}
Intercepting the purchase action
Using the Paywall Action Interceptor to process transactions in paywallObserverMode
Updated about 1 year ago
If you need to use the Paywall Action Interceptor to process transactions with your own subscription infrastructure