Screens display
This sections details how to display a Purchasely screen in a few lines of code
Purchasely screens, also called presentations or paywalls, can be displayed directly via their identifier, but the preferred way is to use a placement.
You can learn more about placements & screens in their dedicated article.
Purchasely will automatically render the view to display, which can be configured entirely remotely from the Purchasely console. The view is rendered with native components, using UI Kit on iOS and View on Android, making it fully compatible with iOS & Android phones, tablets, and TVs.
Display a placement
A placement represents a specific location in your user journey inside your app (e.g. Onboarding, Settings, Home page, Article). A placement is linked to a Screen and a single Screen can be used for different Placements. You can create as many Placements as you want, and this is the only thing that ties the app developer to the marketer.
Placements are configured in the section Placements of the Purchasely Console. From there, you can get the placement ID by clicking on the Copy button.
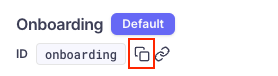
More details about placements in the dedicated page
Once the placements are defined and called from the app, you can change the displayed paywall remotely without any developer action.
let placementId = "<<default_placement>>"
// Get the UIViewController to present
let purchaselyController = Purchasely.presentationController(for: placementId)
// Retrieve the view to display in your layout hierarchy
val presentationView = Purchasely.presentationView(
context = context,
properties = PLYPresentationProperties(
placementId = "<<default_placement>>",
onClose = {
// remove view from layout hierarchy
})
) { result, plan ->
when (result) {
PLYProductViewResult.PURCHASED -> Log.d("Purchasely", "User purchased ${plan?.name}")
PLYProductViewResult.CANCELLED -> Log.d("Purchasely", "User cancelled purchased")
PLYProductViewResult.RESTORED -> Log.d("Purchasely", "User restored ${plan?.name}")
}
}
await Purchasely.presentPresentationForPlacement({
placementVendorId: '<<default_placement>>',
isFullscreen: true,
});
await Purchasely.presentPresentationForPlacement('<<default_placement>>');
private PurchaselyRuntime.Purchasely _purchasely;
_purchasely.PresentPresentationForPlacement(
'<<default_placement>>',
OnPresentationResult,
OnPresentationContentLoaded,
OnPresentationContentClosed,
'contentId',
true
);
Purchasely.presentPresentationForPlacement('<<default_placement>>');
Limitations
Displaying a Screen as described above is synchronous, as such it always returns a View to be displayed. Consequently it carries a few limitations:
- You cannot pre-fetch the Screen
- A loading indicator is displayed until the network call is executed and the view fully rendered
- You cannot deactivate a placement to not display a Purchasely Screen, your default Screen will be displayed
- You cannot display your own screen, your default Purchasely screen will be displayed
To overcome those limitations, you should consider using the asynchronous method that allows you to pre-fetch the presentation and chose not to display a Screen for a specific placement.
More details about the different methods available to display a screens
Close & Callback
Once a successful purchase or restoration has occurred, Purchasely screens will be automatically closed by the SDK, except in Kotlin/Java, where you need to implement the onClose
callback.
For more details on how to retrieve this information or manage it yourself in paywallObserver mode, refer to the next step.
In all other cases, if you want to close the Purchasely presentation, you must do it manually.
// just dismiss the UIViewController returned by Purchasely
val presentationView = Purchasely.presentationView(
context = context,
properties = PLYPresentationProperties(
placementId = "<<default_placement>>",
onClose = {
// The onClose callback to implement
// remove view from layout hierarchy here
})
) { result, plan ->
when (result) {
PLYProductViewResult.PURCHASED -> Log.d("Purchasely", "User purchased ${plan?.name}")
PLYProductViewResult.CANCELLED -> Log.d("Purchasely", "User cancelled purchased")
PLYProductViewResult.RESTORED -> Log.d("Purchasely", "User restored ${plan?.name}")
}
}
Purchasely.closePresentation();
Purchasely.closePresentation();
Purchasely.ClosePresentation();
Purchasely.closePresentation();
Updated 3 days ago