Deeplinks management
The section provided details on how to implement deeplink management with the Purchasely SDK
About Purchasely deeplinks
Purchasely SDK is able to manage deeplinks.
This allows to create no-code deeplink automations and open a Purchasely Screen automatically at the app start by using a simple deeplink.
To enable deeplink management, you first need to complete the App Scheme for your App.
In the Purchasely Console, navigate to App Settings > Store Configuration
Then fill in the App Scheme for each App store.
Universal links (eg: https://purchasely.io instead of ply in the above example) can also be used.
What are Purchasely deeplinks used for?
Purchasely supports the use of Deeplinks to trigger different actions to improve conversion, retention and upsell. You can send a Push or an email with that deeplink and Purchasely will open the requested presentation or page for you.
Here are the actions Purchasely supports:
- Display a paywall
- Display a placement
- Update credit card (Deeplink to App Store)
- Display the user subscriptions
Deeplinks are also used to preview paywalls and screen on your device (directly inside your app) by scanning a QR code displayed in the upper right corner of the Paywall builder inside the Console.
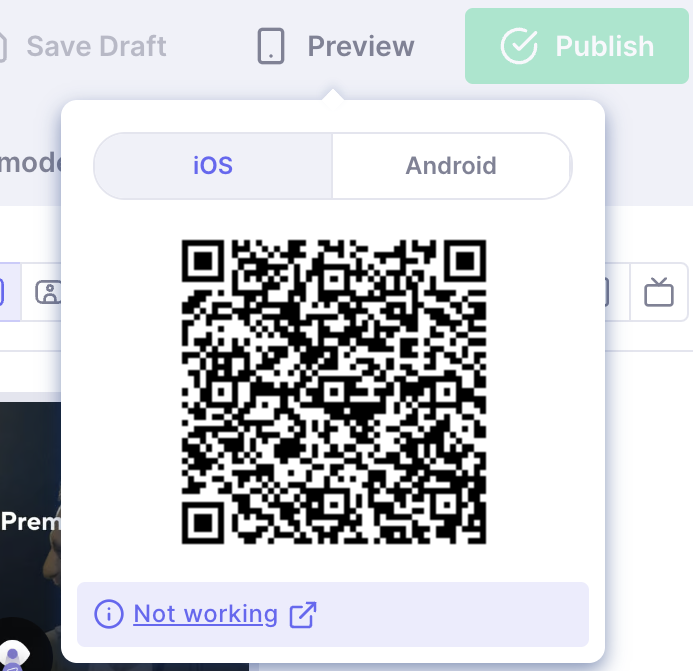
Only a certain type of deeplinks, matching a specific pattern are recognized and handled by Purchasely.
Deeplinks which do not match the pattern are just ignored
Deeplink implementation
To manage deeplinks you need to do 3 things:
- Pass the deeplink to the Purchasely SDK when it is received by the application
- Allow the Purchasely SDK to display content over your interface
- Set a default presentation handler to get the result of what was done by the user on the paywall / screen
1. Passing the deeplink to Purchasely SDK
To enable the Purchasely SDK to analyze the deeplink, the app needs to provide it using the following code:
// ---------------------------------------------------
// If you are **NOT** using SceneDelegate
// ---------------------------------------------------
// AppDelegate.swift
import Purchasely
func application(_ application: UIApplication, open url: URL, sourceApplication: String?, annotation: Any) -> Bool {
// You can chain calls to multiple handler using a OR
return Purchasely.isDeeplinkHandled(deeplink: url)
}
// ---------------------------------------------------
// If you are using SceneDelegate
// ---------------------------------------------------
// SceneDelegate.swift
import Purchasely
func scene(_ scene: UIScene, willConnectTo session: UISceneSession, options connectionOptions: UIScene.ConnectionOptions) {
// …
if let url = connectionOptions.urlContexts.first?.url {
_ = Purchasely.isDeeplinkHandled(deeplink: url)
}
}
func scene(_ scene: UIScene, openURLContexts URLContexts: Set<UIOpenURLContext>) {
if let url = URLContexts.first?.url {
_ = Purchasely.isDeeplinkHandled(deeplink: url)
}
}
class MyActivity : FragmentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
//retrieve intent data to get deeplink that opened your activity
val data = intent.data
if(data != null) {
//Purchasely sdk will return true if it handles the deeplink
val isHandledByPurchasely = Purchasely.isDeeplinkHandled(data)
}
}
}
Purchasely.isDeeplinkHandled('app://ply/presentations/')
.then((value) => console.log('Deeplink handled by Purchasely ? ' + value));
Purchasely.isDeeplinkHandled('app://ply/presentations/')
.then((value) => print('Deeplink handled by Purchasely ? $value'));
// If you grab the deeplink inside your Cordova code you can call
Purchasely.isDeeplinkHandled("app://ply/presentations/", (handled) => {
console.log("Was deeplink handled by Purchasely? " + handled);
});
private PurchaselyRuntime.Purchasely _purchasely;
_purchasely.IsDeeplinkHandled("app://ply/presentations/");
2. Allowing the display
Your app might have a launch routine that requires to be fulfilled before another screen can be displayed. It can be splash screen, on boarding, login, displaying an ad etc...
For that reason, the display of Purchasely deeplinks is deferred until you authorize it.
Once your app is ready, notify the Purchasely SDK by using the following code:
Purchasely.readyToOpenDeeplink(true)
Purchasely.readyToOpenDeeplink = true
Purchasely.readyToOpenDeeplink(true);
Purchasely.readyToOpenDeeplink(true);
Purchasely.readyToOpenDeeplink(true);
_purchasely.SetIsReadyToOpenDeeplink(true);
When should I allow the display of deeplinks?
Your app might have a launch routine that requires to be fulfilled before another screen can be displayed. It can be splash screen, on boarding, login …
The display of Purchasely deeplinks is deferred until you authorize it. Once your app is ready, notify the Purchasely SDK by calling the appropriate method referred on the left.
Supported deeplinks and override display
Updated about 1 year ago