How the paywallObserver mode works?
This section provides details on how the paywallObserver mode works
The paywallObserver
mode allows the use Purchasely on top of an existing subscription infrastructure, such as RevenueCat or an existing in-house subscription platform.
The existing subscription infrastructure continues to process transactions and the Purchasely platform is used on top of it. The Purchasely SDK observes these transactions to fill the Purchasely Dashboards with the data, but it does not acknowledge them with the App stores.
General principles
A typical purchase flow will be as follows:
- the Paywall is displayed by the Purchasely SDK
- when the user clicks on a purchase button, the Purchasely SDK hands over to the app using the Paywall Action Interceptor, providing the app with the references of the plan that was tapped
More details on processing transactions with the Paywall Action Interceptor
More details on using Purchasely with RevenueCat - the app processes the transaction on its own
- either through a 3rd party SDK (like RevenueCat)
- or directly through StoreKit or Google Play Billing
- once processed, the app synchronizes the transaction with the Purchasely SDK by calling the
Purchasely.synchronize()
method - and notifies the Purchasely SDK that the paywall can now be closed
What happens with the transaction processed by my own system?
Once the app has processed the transaction, the Purchasely SDK fetches the receipt created and sends it to the Purchasely Platform, to feed the platform with all the analytics associated to the receipt (subscription events, revenue, mapping with the user etc).
The transaction will however not be finished (App Store) nor acknowledged (Google Play Store). In the Paywall Observer Mode, this is the responsibility of the developer to do it.
The other mode available with the Purchasely SDK is the full
mode.
More details about the full mode
Architecture diagram paywallObserver mode
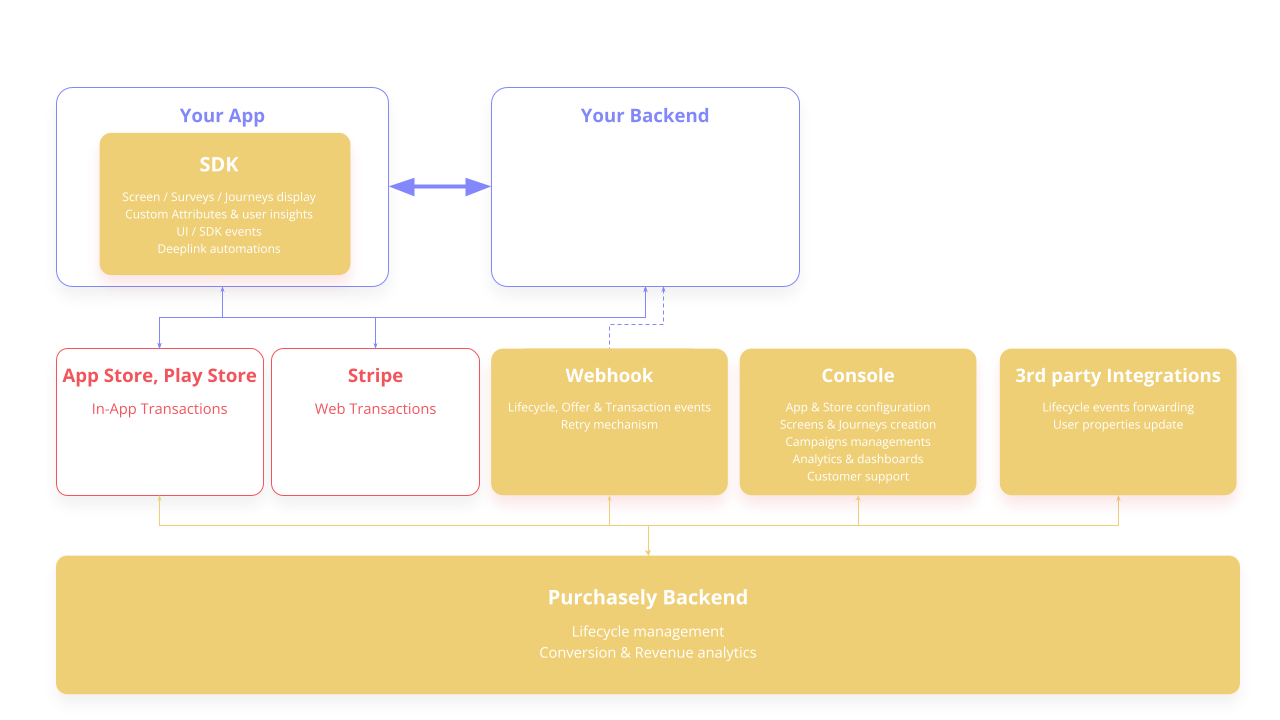
Updated 26 days ago
Start the implementation of the SDK by initializing it in paywallObserver Mode